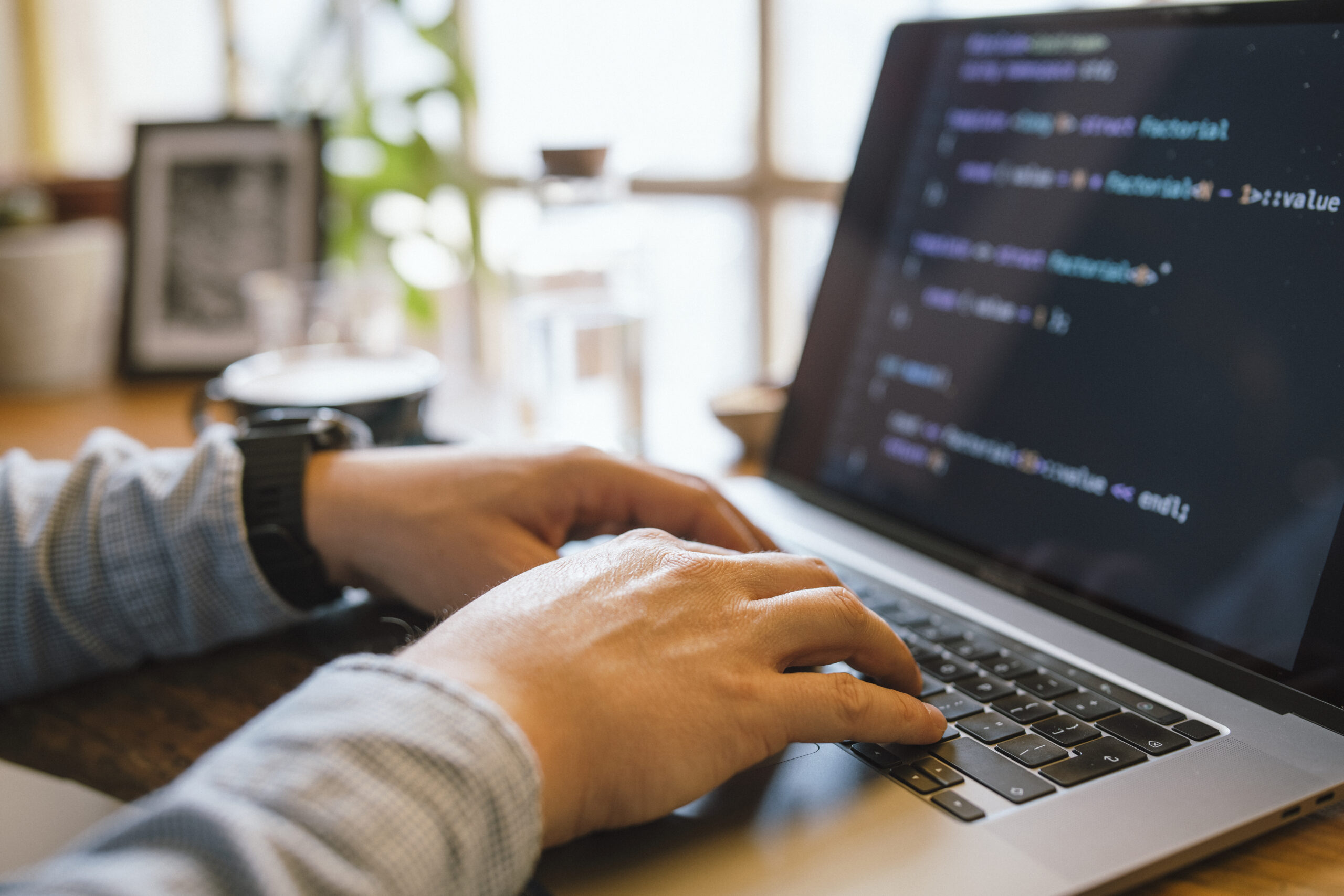
Debugging is Just about the most necessary — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to resolve troubles successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help you save several hours of annoyance and considerably transform your efficiency. Here i will discuss quite a few procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging expertise is by mastering the resources they use every day. When composing code is one particular Portion of development, knowing ways to interact with it proficiently for the duration of execution is equally important. Modern-day progress environments arrive Outfitted with potent debugging abilities — but several developers only scratch the floor of what these resources can do.
Choose, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage by code line by line, and also modify code on the fly. When used effectively, they let you notice exactly how your code behaves all through execution, that's priceless for tracking down elusive bugs.
Browser developer equipment, including Chrome DevTools, are indispensable for front-conclude developers. They allow you to inspect the DOM, watch network requests, perspective true-time effectiveness metrics, and debug JavaScript while in the browser. Mastering the console, sources, and network tabs can transform discouraging UI concerns into workable duties.
For backend or procedure-level builders, applications like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command about running procedures and memory management. Mastering these tools might have a steeper Mastering curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle systems like Git to comprehend code heritage, obtain the precise moment bugs had been launched, and isolate problematic variations.
Ultimately, mastering your resources implies likely further than default configurations and shortcuts — it’s about developing an personal familiarity with your progress ecosystem so that when issues arise, you’re not missing at the hours of darkness. The greater you know your tools, the greater time you can spend fixing the actual issue instead of fumbling via the process.
Reproduce the issue
Probably the most essential — and sometimes disregarded — measures in powerful debugging is reproducing the challenge. Just before jumping in the code or generating guesses, developers need to produce a reliable setting or situation wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Talk to inquiries like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the exact problems underneath which the bug occurs.
When you finally’ve collected plenty of details, try to recreate the challenge in your local ecosystem. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If the issue seems intermittently, consider crafting automated assessments that replicate the sting circumstances or state transitions included. These exams don't just assist expose the challenge but also avoid regressions Sooner or later.
At times, The difficulty may be surroundings-precise — it'd occur only on specified operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital devices, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes safely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages are often the most valuable clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers should find out to treat mistake messages as immediate communications from your method. They often show you just what exactly transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by examining the concept thoroughly and in complete. Lots of developers, especially when underneath time stress, look at the main line and promptly commence making assumptions. But further within the mistake stack or logs might lie the legitimate root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into areas. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or function activated it? These questions can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the error transpired. Check out similar log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These generally precede larger troubles and supply hints about opportunity bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Mastering to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Correctly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you have an understanding of what’s going on underneath the hood without needing to pause execution or step with the code line by line.
A great logging method begins with recognizing what to log and at what level. Typical logging amounts incorporate DEBUG, Data, WARN, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic facts all through progress, Details for normal functions (like profitable commence-ups), WARN for potential concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Stay away from flooding your logs with excessive or irrelevant details. Excessive logging can obscure crucial messages and slow down your process. Give attention to important situations, point out alterations, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Permit you to monitor how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-assumed-out logging strategy, you could reduce the time it requires to identify problems, achieve further visibility into your purposes, and improve the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To successfully detect and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working complex concerns into manageable elements and observe clues logically to uncover the foundation induce.
Start by gathering proof. Look at the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys a crime scene, collect just as much applicable details as you'll be able to with no jumping to conclusions. Use logs, check circumstances, and user reports to piece together a clear photograph of what’s happening.
Subsequent, form hypotheses. Ask yourself: What can be producing this habits? Have any alterations just lately been created on the codebase? Has this situation occurred prior to under identical situation? The aim would be to slender down options and discover probable culprits.
Then, take a look at your theories systematically. Endeavor to recreate the challenge within a controlled ecosystem. In case you suspect a particular function or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and let the final results direct you nearer to the reality.
Pay out close attention to smaller information. Bugs often cover inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the actual issue, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for potential difficulties and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique challenges methodically, and turn out to be simpler at uncovering concealed issues in sophisticated devices.
Write Tests
Creating assessments is among the simplest ways to enhance your debugging techniques and overall improvement efficiency. Exams not merely enable capture bugs early but will also function a security Web that offers you self confidence when building variations towards your codebase. A well-tested software is much easier to debug because it allows you to pinpoint precisely exactly where and when an issue happens.
Begin with unit exams, which give attention to personal features or modules. These little, isolated tests can rapidly reveal whether or not a specific piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Unit checks are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in sophisticated methods with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Feel critically regarding your code. To check a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. Once the check fails continually, you are able to center on correcting the bug and observe your take a look at pass when The difficulty is resolved. This strategy makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a annoying guessing activity right into a structured and predictable procedure—supporting click here you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution after Answer. But Just about the most underrated debugging equipment is just stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are much too near the code for much too extensive, cognitive exhaustion sets in. You would possibly get started overlooking evident glitches or misreading code you wrote just several hours previously. On this condition, your brain gets considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily through more time debugging sessions. Sitting down before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a little something unrelated to code. It might truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically results in speedier and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It gives your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you face is a lot more than just A brief setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable in the event you make time to mirror and analyze what went Incorrect.
Start off by inquiring on your own a handful of vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater procedures like device screening, code opinions, or logging? The responses often reveal blind places in the workflow or understanding and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your peers is often Specially effective. Whether or not it’s via a Slack concept, a brief create-up, or A fast expertise-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary portions of your advancement journey. In fact, several of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your talent set. So up coming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more successful, confident, and capable developer. The following time you happen to be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.